CoffeShop cz. 1 – Początek projektu
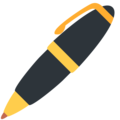
Założyłem pustą Solucje i dodałem do niej poszczególne projekty reprezentujące warstwy aplikacji wraz z powiązaniami żeby móc wykorzystać Dependency Injection.
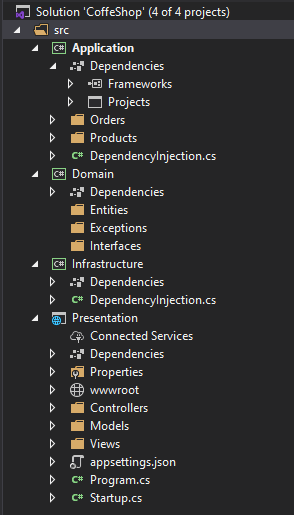
Na poczatek chciałbym zrobić proste API z użyciem MediatR i CQRS do pobierania zmockowanych zamówień i produktów.
using MediatR; using Microsoft.AspNetCore.Http; using Microsoft.AspNetCore.Mvc; using Microsoft.Extensions.DependencyInjection; namespace Presentation.Controllers { [Route("api/[controller]")] [ApiController] public class BaseController : ControllerBase { private IMediator _mediator; protected IMediator Mediator => _mediator ??= HttpContext.RequestServices.GetService<IMediator>(); } }
Dzięki załączeniu Atrybutów Route i ApiController w tej klasie nie ma potrzeby przepisywac ich do klas pochodnych ponieważ są dziedziczone 😀
Wszystkie Command i Queries będą umieszczone w warstwie aplikacji.
Poniżej prezentuje GetAll dla zmockowanych produktów.
using Domain.Entities; using MediatR; using System.Collections.Generic; using System.Threading; using System.Threading.Tasks; namespace Application.Products.Query.GetAllProductsQuery { public class GetAllProductsQuery : IRequest<List<Product>> { public class GetAllProductsQueryHandler : IRequestHandler<GetAllProductsQuery, List<Product>> { public async Task<List<Product>> Handle(GetAllProductsQuery request, CancellationToken cancellationToken) { var products = new List<Product> { new Product { Id = 1, Name = "Cappuccino S", Price = 5.99m, Quantity = 0 }, new Product { Id = 2, Name = "Cappuccino M", Price = 8.99m, Quantity = 0 }, new Product { Id = 3, Name = "Cappuccino L", Price = 9.99m, Quantity = 0 }, new Product { Id = 4, Name = "Espresso", Price = 4.99m, Quantity = 0 }, new Product { Id = 5, Name = "Espresso Doppio", Price = 5.99m, Quantity = 0 }, new Product { Id = 6, Name = "Americano S", Price = 4.99m, Quantity = 0 }, new Product { Id = 7, Name = "Americano M", Price = 6.99m, Quantity = 0 }, new Product { Id = 8, Name = "Americano L", Price = 8.99m, Quantity = 0 } }; return products; } } } }
Kontroler:
using System; using System.Collections.Generic; using System.Linq; using System.Threading.Tasks; using Application.Products.Query.GetAllProductsQuery; using Domain.Entities; using Microsoft.AspNetCore.Http; using Microsoft.AspNetCore.Mvc; namespace Presentation.Controllers { public class ProductsController : BaseController { [HttpGet] public async Task<List<Product>> GetAll() { return await Mediator.Send(new GetAllProductsQuery()); } } }
Teraz wszystko działa kiedy przejdziemy pod <a rel=”noreferrer noopener” href=”https://localhost:https://localhost:<port>/api/products, ale zawsze można lepiej. Dlatego zainstalujemy pakiet Swashbuckle Swagger pamiętając że powinien być on dostępny jedynie w środowisku Dev 😀
Po przejściu pod … ukazuje się
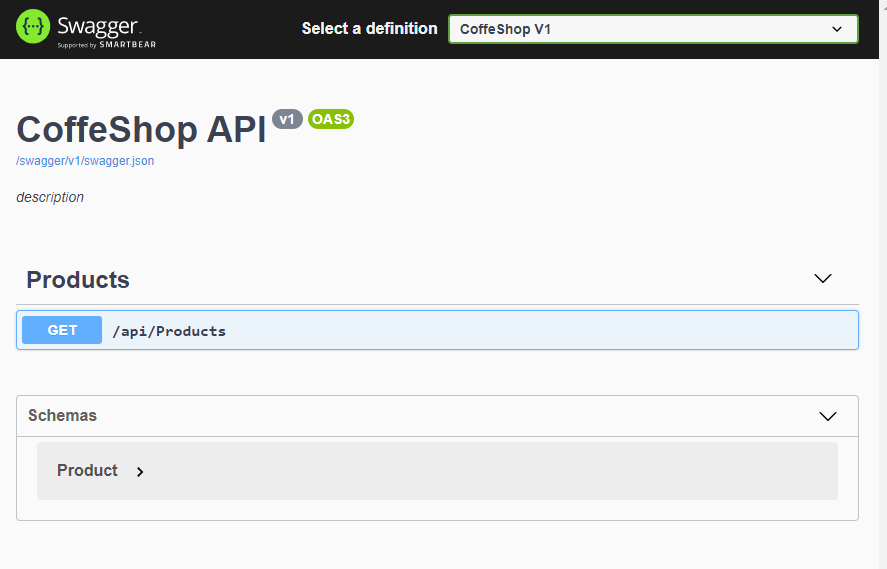
Otwieramy interesujący nas endpoint i w niego strzelamy 😀
Dostajemy odpowiedź wraz z listą dostępnych kaw.
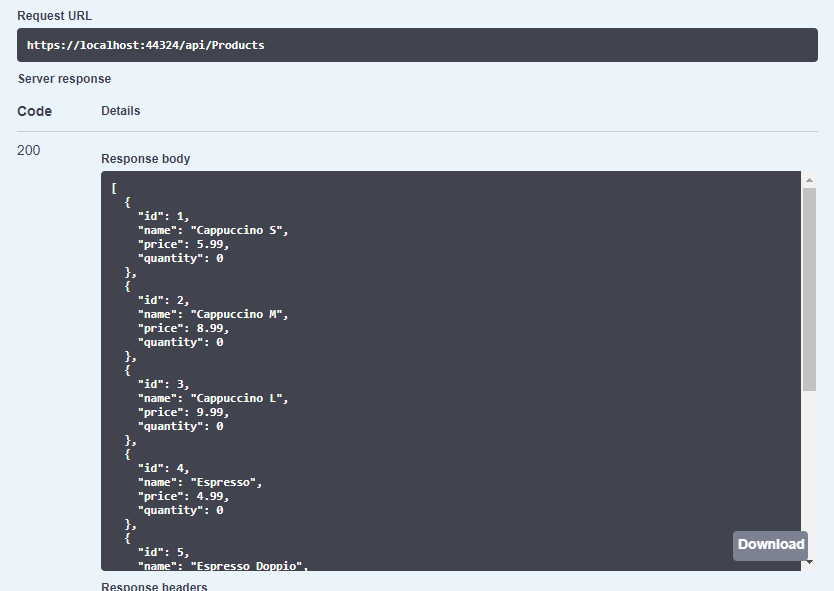
Cały kod zamieszczam na github: https://github.com/MichaelStett/CoffeShop
Dziękuje za Twoją uwagę i do następnego 😀